Plot 3D images
You can plot surfaces from freesurfer:
surf = Surf(surf_path)
v = Viz3()
v.add_surf(surf)
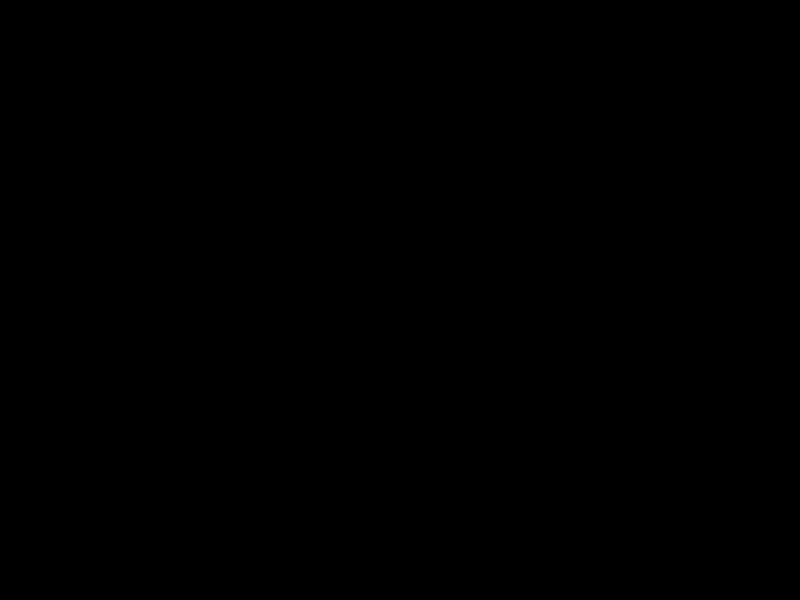
and you can plot the color for the whole surface:
surf = Surf(surf_path)
v = Viz3()
v.add_surf(surf, color=(.5, .5, 0))
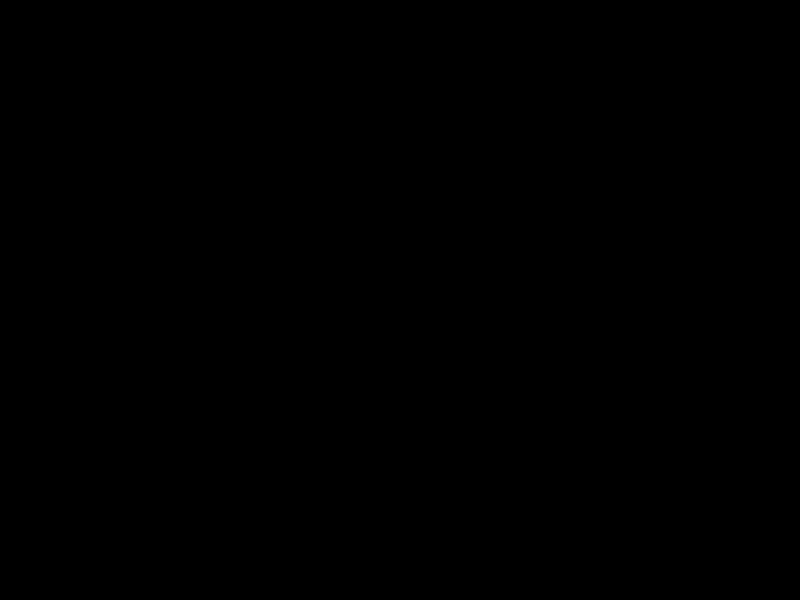
or you can give the value for each vertex.
You can specify the min and max limits with limits_c
.
surf = Surf(surf_path)
v = Viz3()
v.add_surf(surf, values=surf.vert[:, 2], limits_c=(0, 50))
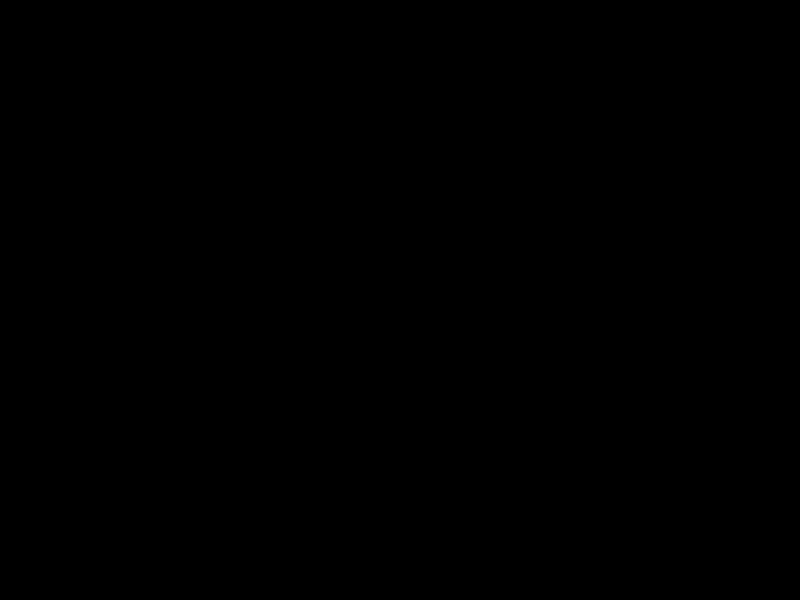
You can add a colorbar at the back of the head (you might need to zoom out to see it):
surf = Surf(surf_path)
v = Viz3()
v.add_surf(surf, values=surf.vert[:, 2], limits_c=(0, 50), colorbar=True)
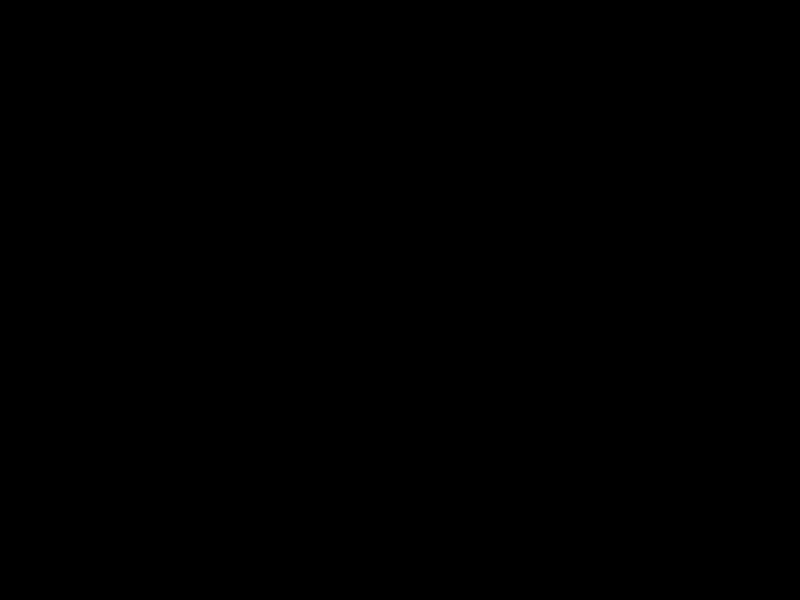
You can then add channel positions, such ECoG on the surface:
surf = Surf(surf_path)
channels = Channels(chan_path)
v = Viz3()
v.add_chan(channels)
v.add_surf(surf, alpha=.8)
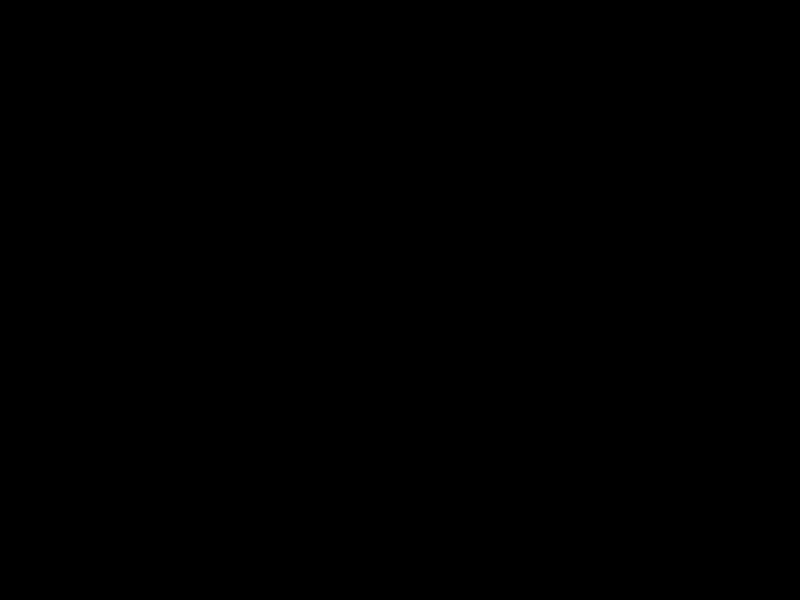
Note
Note how the channel groups had different colors. This is based on the channel labels.
You can also specify one color (in RGB, values between 0 and 1) to use the same color for all the channels:
surf = Surf(surf_path)
channels = Channels(chan_path)
v = Viz3()
v.add_chan(channels, color=(1, 0, 0))
v.add_surf(surf, alpha=.5)
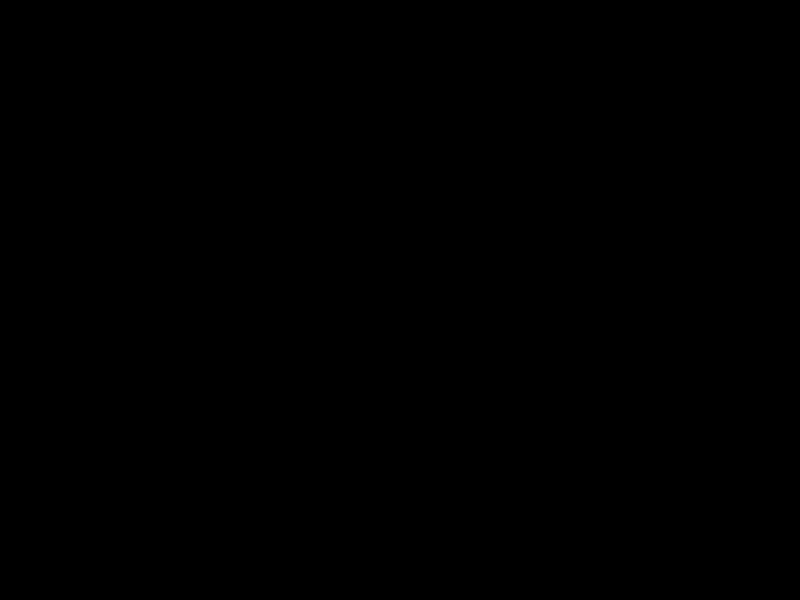
To specify the transparency, enter the keyword alpha
(0: transparent, 1: opaque).
surf = Surf(surf_path)
channels = Channels(chan_path)
v = Viz3()
v.add_chan(channels, color=(1, 0, 0), alpha=0.5)
v.add_surf(surf, alpha=.5)
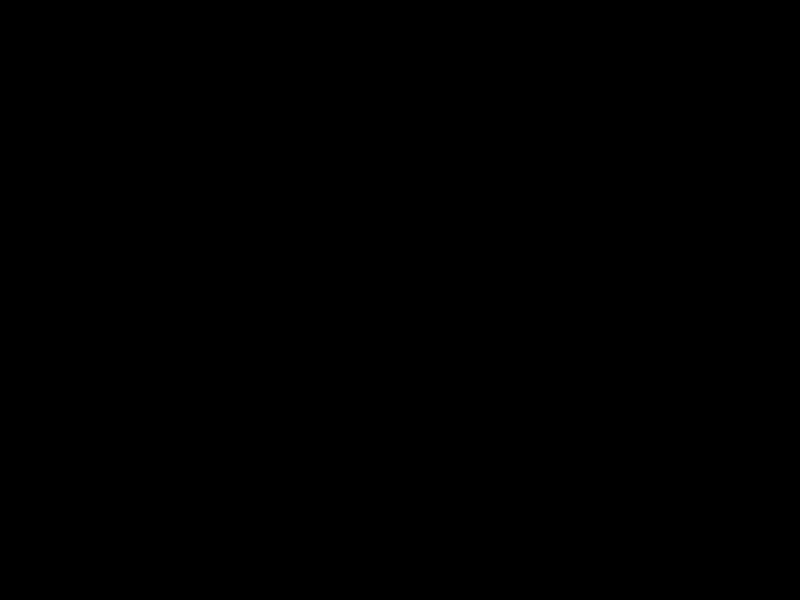
You can also specify the color for each channel (in this example, we alternate red and blue channels).
from numpy import tile
surf = Surf(surf_path)
channels = Channels(chan_path)
color_everyother = tile([[1, 0, 0], [0, 0, 1]], (14, 1))
v = Viz3()
v.add_chan(channels, color=color_everyother)
v.add_surf(surf, alpha=.5)
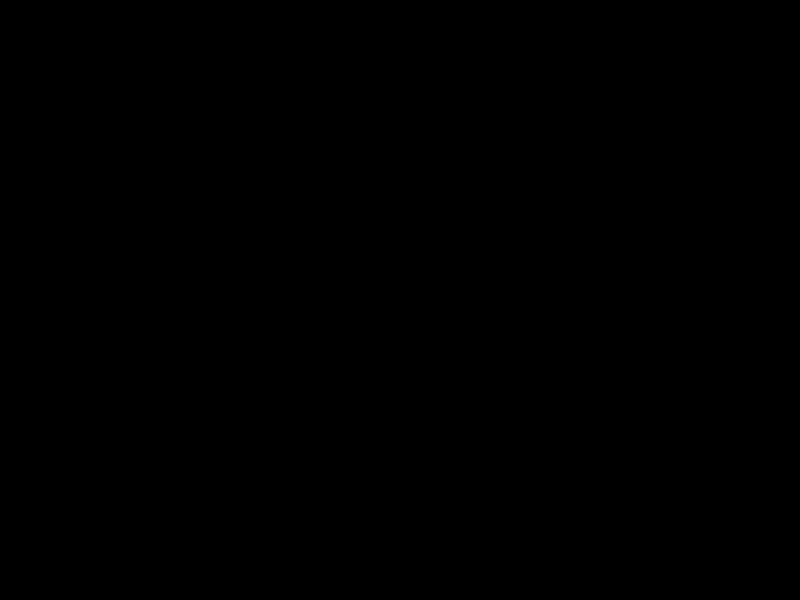
Finally, you can specify the values for each channels (and add a colorbar).
from numpy import arange
surf = Surf(surf_path)
channels = Channels(chan_path)
v = Viz3()
v.add_chan(channels, values=arange(channels.n_chan), colorbar=True)
v.add_surf(surf, alpha=.5)
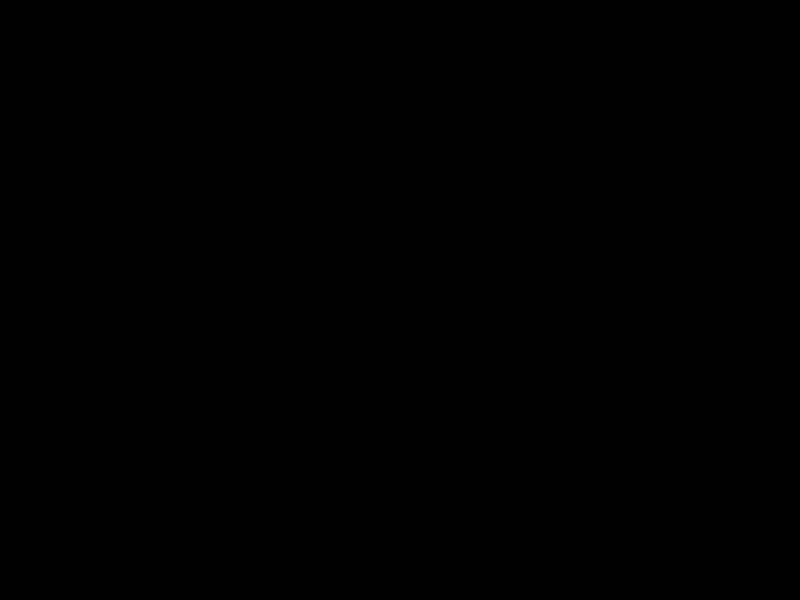